Getting to know $watch
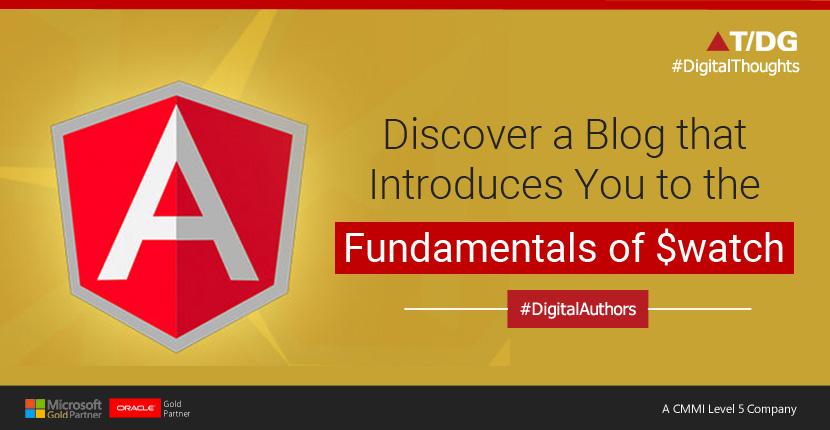
This week I came across a $watch, and thought I’d share what I had learnt about it. First, you need to understand how the Angular digest cycle works.
The Digest Cycle in AngularJS
The cycle is where Angular checks to see if there are any changes to all the variables under all the $scopes. Lets say you have a variable, $scope.TDG , defined in your controller and this variable was marked for being watched, then you are implicitly telling Angular to monitor the changes on TDG in each iteration of the loop. Obviously not all the variables attached to scope are being watched, that would not be very efficient. There are two ways of declaring a $scope variable as being watched.
- By using it in your template via expression. ie. {{TDG}}
- By adding it manually via $watch service
Implementing $watch
Method # 1 : Scope variables used in the expression {{TDG}} have $watch created for them. This is the most common scenario. Using Angular directives such as ng-repeat also create implicit watches.
Method # 2: $watch service helps you to run some code when some value attached to the $scope has changed. Here is small example.
Use-case: We wanted to prevent the use from using white spaces in the creation of a name. In order to prevent the user from added spaces we used a $watch function and replace the white spaces
function MyController($scope) {
$scope.$watch('name', function () {
$scope.name = $scope.name.replace(/\s+/g, '');
});
}
Now we have a input with ng-model name as ‘name’. Whenever the user tries to type a space, it is immediately replace with nothing, giving the effect of not being able to add spaces.
Creating your own $watch function
When you register a watch you pass two functions as parameters to the $watch() function:
- A value function
- A listener function
$scope.$watch(function1() {}, function2() {} );
The first function is the value function and the second function is the listener function. The value function should return the value which is being watched. AngularJS can then check the value returned against the value the watch function returned the last time. That way AngularJS can determine if the value has changed.
That’s it ! Hope this helps you when you need to register your own $watch. Good luck and happy coding !