Using Controller-as-a syntax and why it’s important
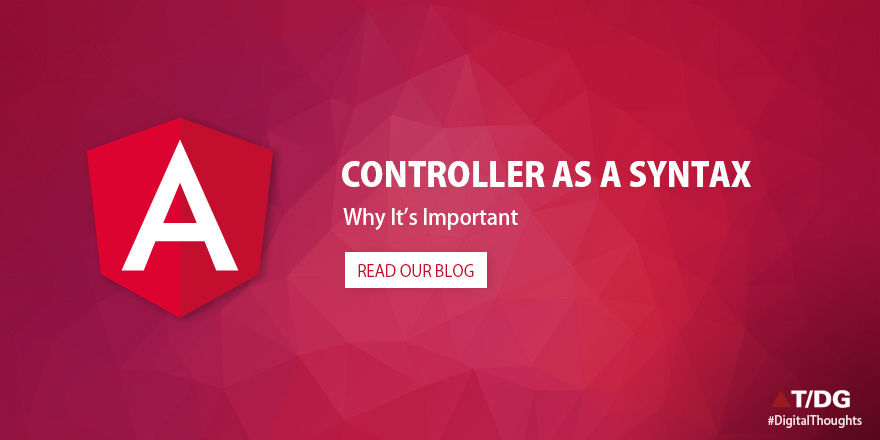
What is “controller as a” syntax ?
It’s a new way of defining your controller. Instead of using $scope, we can use the ‘this‘ in its place.This helps with the architecture of the controller, having a clearer scoping and smarter controllers. This change specifically came about after the 1.2 release of AngularJS.
Why should I start using Controller as a syntax ?
AngularJS 2.0 is being talked about quite a bit. One of the important discussion with AngluarJS 2.0 is how it will be quite different from the current version.
Angular 2.0. It is essentially a re-imagining of AngularJS for the modern web — Rob Eisenberg, Angular 2.0 core team.
One of the major changes will be removing $scope. The concept of scope will still remain, but it will be bounded to directives. Now would be a good time to understand of how “controller as a syntax” works, so that when the changes comes around you will be ready.
How to do I use this syntax ?
Typically you would define your controller as such:
app.controller('MainCtrl', function ($scope) {
$scope.companyName = 'The Digital Group';
});
To use the new syntax, you would remove scope and define the controller in this fashion:
app.controller('MainCtrl', function () {
var vm = this;
vm.companyName = 'The Digital Group';
});
and the way you declare your controller in the view would be :
<div ng-controller="MainCtrl as main">
{{ main.company }}
</div>
This helps in cleaning up the code, now we done have floating variables such as {{company}}, we know the variable company is defined in MainCtrl.
Lets take another example where we have multiple controllers. Take for instance this situation where we have three different nested controller :
<div ng-controller="MainCtrl">
{{ company }}
<div ng-controller="SomeCtrl">
Scope company: {{ company }}
Parent company: {{ $parent.company }}
<div ng-controller="SomeOtherCtrl">
{{ company }}
Parent company: {{ $parent.company }}
Parent parent company: {{ $parent.$parent.company }}
</div>
</div>
</div>
and now take a look at this same code, but done using controller as a syntax:
<div ng-controller="MainCtrl as main">
{{ main.company }}
<div ng-controller="SomeCtrl as some">
Scope company: {{ some.company }}
Parent company: {{ main.company }}
<div ng-controller="SomeOthertherCtrl as someOther">
Scope company: {{ someOther.company }}
Parent company: {{ some.company }}
Parent parent company: {{ main.company }}
</div>
</div>
</div>
Reading this makes it much more easier to understand what the developer was trying to do, and this is how controller as a syntax helps.
In Conclusion, the reason you should use “controller as a syntax” is so that you can make your code more efficient, maintainable, and readable. Also, the fact that the current way of using $scope in your controller won’t exist by the time AngularJS 2.0 rolls aournd.