Understanding Filters in ASP. Net MVC
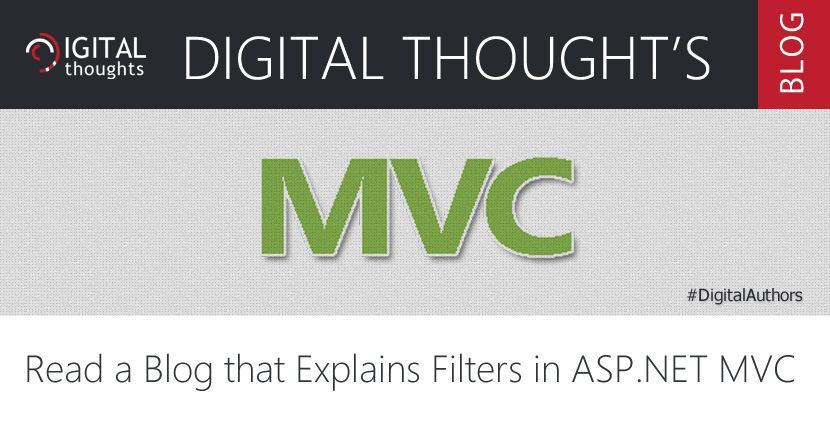
Filters in ASP.net MVC
Filters are interesting and somewhat unique part of ASP.net MVC. Filters are designed to inject logic in between MVC request life cycle events. Filters provide developers with powerful ways to inspect, analyze, capture and instrument several things going around within MVC application. Understanding when each of the filters kick in and play the role can greatly enhance the way developers write logic. plus it gives a very interesting perspective to understand how filters run at multiple points in the request life cycle and have the power to control further execution or prevent request from executing futher. As of MVC5, there are 5 types of filters.
Authentication filters
Authentication filters are new addition from MVC 5. These filters kick in first in the request life cycle and perform the authentication logic.
Authorization filters
Authorization filters are executed after the Authentication filters successfully executed and authorizes users roles to ensure current user has access to request resource.
Action filters
Action filters are executed next in the request life cycle and execute any custom logic you may want to perform before and after action execution.
Result filters
Result filters are executed before and after result execution.
Exception filters
Finally, the exception filters are executed when there is an exception during processing of request.
following diagram depicts when each of the filter fires in the MVC request life cycle.
as you can see from the below diagram, as soon as the controller starts execution through Action Invoker, Authentication and authorization filters are the very first filters to be triggered, followed by model binding which maps request and route data to action parameters. which is then followed by Action filter execution, OnActionExecuting fires before the action has been executed and OnActionExecuted is fired after te Action method has finished execution. last comes the Result filters, OnResultExecuting gets fired before executing result, and OnResultExecuted gets fired after the result has been executed. exception filter are special filters in the sense that they get fired as soon as an exception is thrown and you can write your custom logic to log exception or redirect user to a error page and take appropriate action in this filter.
Filters in Action
Like any other life cycle events of MVC, implementing filters in MVC is very easy.
Authentication Filter
lets start with Authentication Filter, this filter is recent addition to MVC 5, there is no way you would be able to implement this filter in your older apps. you can leverage this filter for performing authentication related stuff. in the request life cycle, after the URL Routing module has selected the route and controller have been initialized, the first thing that kicks off in the Action method execution is Authentication Filter.
To implement Authentication Filter, you must implement IAuthenticationFilter interface along with deriving from FilterAttribute base class. the key here is IAuthenticationFilter interface that lets you implement two methods, OnAuthentication and OnAuthenticationChallenge. OnAuthentication is primarily used for setting identity Principle or any other type of authentication that your application understands, OnAuthenticationChallenge is the special methods which gets executed just before the result execution. the reason might be that as a developer you would want to do some checks here like authorization or redirecting user to a different login page in case of a HttpUnauthorisedResult
case. OnAuthenticationChallenge is used to add a “challenge” to the result before its returned to the user. here is how you would implement it:
don’t worry about “StoreInSession” method call for now, its just logging the events in the session, we will see the output at the end of this to understand what its doing.
Action Filters
Action Filters are the filters that gets executed before and after a method, you can use this filter to implement instrumentation like action performance and other things. to implement Action filter, you need to implement IActionFilter interface and derive from FilterAttribute base class. IActionFilter gives you two methods to implement, OnActionExecuting and OnActionExecuted,. OnActionExecuting gets executed before the Action mehtod execution and OnActionExecuted gets fired after the action method execution.
Authorization Filter
Authorization filter has been in use for performing authentication and other stuff prior to Authentication filters. to implement Authorization Filter you need to implement IAuthorizationFilterinterface and derive from FilterAttribute base class. the interface IAuthorizeFilter gives you one method to implement, “OnAuthorization” where you can perform authorization related checks.
Result Filter
Result filter are the filters that gets executed before and after executing result to a particular http request. you can leverage these filters to do various checks like instrumentation, or manipulating results before they are sent back to browser etc. to customize Result filter, implement IResultFilter interface along with deriving from FilterAttribute class.
Exception Filter
Exception filters are special filters in the sense that they occur when there is an exception in request execution. you can implement and customize this filter to catch exception as it occurs and probably log it to file system or database and then redirect use to a error page or take any other action that suits your need. to customize this filter implement IExceptionFilter and derive from FilterAttribute base class.
finally, once you have customized all your filters, you would need to go and tell MVC framework to hook up your filters in the request life cycle. this process is called as registration of filters in MVC life cycle. just by implementing some interfaces doesn’t lead to automatic discovery of new customized version of filter to take into effect.
Registering filters could be done in two ways, the first one is to put as controller or action attributes, but in that case you would need to remember putting it on all the controller or actions. another way of registration is to register at a global level, that way you are assured that it will executed for all the controller s and actions.
for option#2, go to Global.asax.cs and under Application_Start event register the customized filters as follows.
that’s it. once you have registered your filters MVC framework will take care of invoking them through out the life cycle.
Coming back to output of capturing events and logging details in session.
refer to the attached source code, link is available at the end of this blog. in a nutshell, all I have done in the source code is to implement all the filters and adding a log entry into the Session to see which ones gets fired and the order of it. ( just to validate my diagram at the beginning ).
the output of the application is as follows, as you can see OnAuthentication is fired first, followed by OnAuthorization, then Action fitlers (OnActionExecuting and OnActionExecuted), followed by OnAuthenticationChallenge ( which I told earlier that it will be fired before executing results), followed by Result filters (OnResultExecuting and OnResultExecuted ). you might be wondering why OnResultExecuted is not shown here? that because OnResultExecuted is fired after sending result back to browser, i.e. we see this page and that concludes our request.
Final Thoughts
MVC is very flexible and robust framework, and Microsoft has written this framework with lot of thinking in mind. custom filters are great example of MVC’s features. use these filters as and when required and leverage one of best framework by Microsoft.
Link to Source code
https://github.com/chetanvihite/FiltersinMVC2
As always, feedback, suggestion or comments are always welcome!
Happy Coding.