Locator Strategies in Selenium WebDriver : CSS selectors
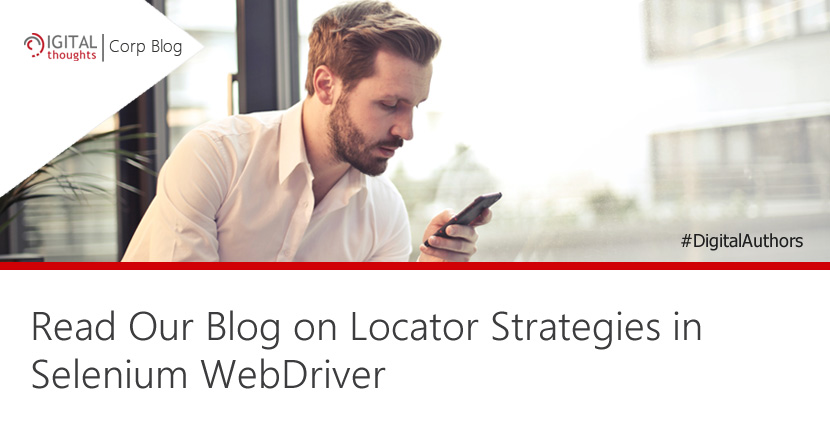
Selenium Locators: Locate element by CSS selector using html tag attributes
This a fall back when all options fail, you can use parent child relation in tags, in case you need to use complex strategy to locate elements. CSS selectors are string representation of HTML tags, attributes, Id, Class. It’s somewhat complex strategy compared to the previous we seen. But we can locate the elements which don’t have even Id or name or class using CSS selectors.
We can use different combinations of attributes to locate an element using CSS selector.
- Tag and ID
- Tag and class
- Tag and attribute
- Tag, Id and attribute
- Tag, class, and attribute
- nth-child()
- Inner text (Not supported by WebDriver)
These are commonly used combinations, for more combinations you can refer this
URL: http://www.w3schools.com/cssref/css_selectors.asp
But you need to check first that the combination you are going to use is supported by Selenium WebDriver.
Tag and ID
In this case you need to follow this syntax css=tag#id. For Id we need to use # sign before id value.
Example:
<input id = "txtName" class = "textboxcss" tabindex = "1" type = "text" >
css = input# txtName
WebElement cssele = driver.findElements(By.cssSelector("input#txtName"));
Here input is tag name and Id is txtName with # sign.
Tag and Class
In this case you need to follow this syntax css=tag.classname. For class we need to use dotbefore class value. If there is space between classname like classname you need to use dot in between space.
Example 1:
<input id="txtName" class="textboxcss" tabindex="1" type="text">
css=input.textboxcss
WebElement cssele = driver.findElements(By.cssSelector("input.textboxcss"));
Example 2:
<input id="txtName" class="textboxcss top" tabindex="1" type="text">
css=input.textboxcss.top
WebElement cssele = driver.findElements(By.cssSelector("input.textboxcss.top"));
Here input is tag name followed by dot and class name textboxcss. In example 2 class name is
textboxcss<space>top in that case we put dot in between textboxcss and top.
Tag and Attribute
In this case when we don’t have both Id or class name we go for html attributes given in tags.
Syntax for this combination is css=tag[attribute=’value’]. We need to use square brackets to specify the attribute and its value. Put the value between single quotes when you are writing script in Java.
Example:
<input value="Reading" type="checkbox">
css=input[type=’checkbox’]
or
css=input[value=’Reading’]
Here in first case type is attribute and checkbox is its value, in other case value is an attribute and its value is Reading.
Tag, ID and Attribute
In this case when we have common id but other attributes are different, we go with this combination. Syntax for this combination is css=tag#id[attribute=’value’].
Example:
<input id="txtName" class="textboxcss" tabindex="1" name="taComment" type="text">
<input id="txtName" class="textboxcss" tabindex="1" name="tbComment" type="text">
css=input#txtName[name=’taComment’]
WebElement cssele = driver.findElements(By.cssSelector("input#txtName[name=’taComment’]"));
Here for both textboxes id is same ever class name is also same, only name is different for both. So if we want to locate first text box we will go with locator given in above example. For second text box we need to change value of name attribute in same combination.
Tag, Class and Attribute
In this case when we have id but we have class name which is common around other elements but other attributes are different, we go with this combination. Syntax for this combination is css=tag.classname[attribute=’value’].
Example:
<input class="textboxcss" tabindex="1" name="taComment" type="text">
<input class="textboxcss" tabindex="1" name="tbComment" type="text">
css=input.textboxcss [name=’taComment’]
WebElement cssele = driver.findElements(By.cssSelector("input.textboxcss [name=’taComment’]"));
nth-chilld()
In this case we have same Id or class name and other attributes for different elements, we can go with nth-child().
Syntax for this combination is css=tag:nth-child(n). Here in syntax we can use any combination discussed above. With that we need to use: nth-child(n). n represent child number.
Example:
<ul>
<li>C</li>
<li>C++</li>
<li>C#</li>
<li>Java</li>
<li>Python</li>
<li>Ruby</li>
</ul>
css= li:nth-child(n)
WebElement cssele = driver.findElements(By.cssSelector("li:nth-child(n)"));
Here if we can see the ul li parent child structure. We have only tag names which are common to everyone. Here if we want to locate sat Java we will put n=4 in above command.
Inner text
This is right now not supported by WebDriver in case of CSS, but most probably will support in upcoming Selenium 3 or 4.
Syntax: css= tag:contains(‘inner text’), Here in syntax we can use any combination discussed above. With that we need to use (:) contains(inner text).
Example:
<span>Upload you pic :</span>
css= span:contains(‘Upload you pic ‘)
WebElement cssele = driver.findElements(By.cssSelector("span:contains(‘Upload you pic‘)"));
Absolute and Relative Path
The Examples we have seen till now are related to only single tag and its attribute. But when we require to build path using parent child relation we need to give its either absolute or relative path.
Example:
<div>
<ul>
<li>C</li>
<li>C++</li>
<li>Python</li>
</ul>
</div>
Consider here we want to locate Python using parent child relation. In that case relative path will be
Relative path: css=div<space>ul<space>li:nth-child(3) or css=div<space>li:nth-child(3). In second combination we have removed ul. Space denotes it’s a Relative path. Here WebDriver will search 3rd li inside given div and ul.
And if we want Absolute path for same, it will be
Absolute path: css= div>ul >li:nth-child(3) or css=ul> li:nth-child(3). Here angular bracket denotes Absolute path. It’s an exact path for given element.
To know the difference between both let’s consider the example.
Someone asked you where is your office? Most common answer is Hinjewadi. But if a courier boy asked you the address you will tell full and exact address of your office. 1st one is relative path to your office but 2nd is absolute one.
We will continue with Xpath selectors in next week’s blog.
Write a comment
- afiah September 22, 2018, 5:48 amThanks you for sharing this unique useful information content with us. Really awesome work. keep on bloggingreply
- Sai September 15, 2018, 12:37 pmYou got an extremely helpful website I actually have been here reading for regarding an hour.reply
- Sri Venky September 14, 2018, 11:51 amInspiring writings and I greatly admired what you have to say , I hope you continue to provide new ideas for us all and greetings success always for you..Keep update more information..reply
- Gowsalya September 10, 2018, 11:16 amYour good knowledge and kindness in playing with all the pieces were very useful. I don’t know what I would have done if I had not encountered such a step like this.reply
- pooja saravanan September 4, 2018, 7:53 amAwesome article. It is so detailed and well formatted that i enjoyed reading it as well as get some new information too.reply
- Rohit Sakhawalkar February 9, 2017, 2:53 pmYes surely we can, if you share the code snippet, I might able to help you.reply
- Sudha Raman February 9, 2017, 1:23 pmuseful and very clear information about CSS selector. i have a query about identifying the web images. will it possible to use CSS selector for finding web images. for selenium hands on workout check out <a href="http://www.credosystemz.com/training-in-chennai/best-selenium-training-in-chennai/" rel="nofollow">selenium training in chennai</a>reply
- Nollie October 23, 2016, 10:35 amNone can doubt the veacirty of this article.reply
- harish March 10, 2016, 4:34 pmThis information is impressive..I am inspired with your post writing style & how continuously you describe this topic. <a href="http://www.traininginsholinganallur.in/selenium-training-in-chennai.html" rel="nofollow"> Selenium training in chennai</a>reply
- Rohit Sakhawalkar November 17, 2015, 12:45 pmYou are most welcome :)reply
- amar November 17, 2015, 11:51 amThanks Rohit. I see you already mentioned that 'contains' is not supported for css selectors. I should have read thoroughly. Thanks for sharing all the info.reply
- Rohit Sakhawalkar November 14, 2015, 8:17 amAmar as I mentioned earlier its not supported in current version of selenium (2.0), but if you are following selenium community selenium 3.0 is about to release and selenium 4.0 beta version is also released for which they are planning to add more css keywords like contains which will make out life easy. So for future use I added it :). In case of selenium IDE it does support in element identification. ANW thanks :). Keep scriptingreply
- amar November 14, 2015, 6:42 amI don't believe contains works in CSS selectors. Like you mentioned css= span:contains(‘Upload you pic ‘) As of today css doesn't support this. This was proposed for css selectors but abandoned years ago. Good rest of the info though.reply
- Rohit Sakhawalkar October 8, 2015, 4:31 pmThank youreply
- Snehal Bahulekar October 8, 2015, 11:24 amNice Article...!!reply
- Rohit Sakhawalkar September 24, 2015, 10:00 amThank you!!!reply
- Rohit Jain September 24, 2015, 12:12 amvery nice article and well supported by easy to understand examples.reply
- Rohit Sakhawalkar September 23, 2015, 5:33 pmThank you sirji!!reply
- Rohit Sakhawalkar September 23, 2015, 5:33 pmThank you sirji!!reply
- Abhishek September 23, 2015, 5:12 pmGOOD One. Keep going.reply
- Naveen Shrivastava September 23, 2015, 2:02 pmVery Nice Description and also very excellent stuff's for beginners. Continue this blog till advanced level for others benefit.reply
- rohits September 23, 2015, 12:48 pmThank you Vijayreply
- vijay September 23, 2015, 10:58 amGood information about CSS selectors.Thanks.reply