Test Driven Development by Example
Test-driven development (TDD) is a software development. First the developer writes an (initially failing) automated test case that defines a desired improvement or new function, then produces the minimum amount of code to pass that test, process that relies on the repetition of a very short development cycle and finally refactors the new code to acceptable standards.
The following sequence of steps is generally followed:
- First, the developer writes some (initially failing) tests.
- The developer then runs those tests and they fail because none of those features are actually implemented.
- Next, the developer actually implements those features in the code.
- If the developer writes his code well, then the in next stage he will see his tests pass.
- The developer can then refactor his code, add comments, clean it up.
- The cycle can just continue as long as the developer has more features to add. A flowchart is given below of this cycle
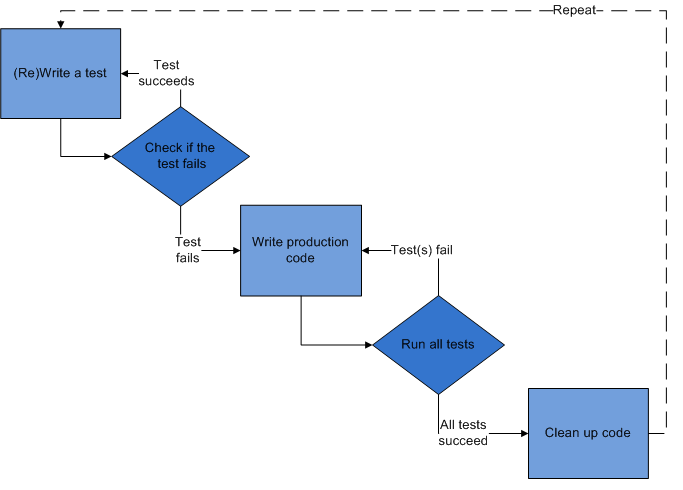
1. 0! should return 12. 1! should also be 13. 2! should return 24. 4! should return 245. 10! should return 3628800
import static org.junit.Assert.*;
import com.tdg.tdd.demo.*;
import org.junit.Test;
public class CalculateFactorialTest {
private FactorialCalculator factCalc=new FactorialCalculator();
@Test
public void shouldReturnOneWhenZeroIn(){
assertEquals(1, factCalc.getFactorial(0));
}
@Test
public void shouldReturnOneWhenOneIn(){
assertEquals(1, factCalc.getFactorial(1));
}
@Test
public void shouldReturnTwo() {
assertEquals(2, factCalc.getFactorial(2));
}
@Test
public void shouldReturnSix() {
assertEquals(6, factCalc.getFactorial(3));
}
@Test
public void shouldReturnTwentyFour() {
assertEquals(24, factCalc.getFactorial(4));
}
@Test
public void shouldFindCorrectFactorialFor10() {
assertEquals(3628800, factCalc.getFactorial(10));
}
}
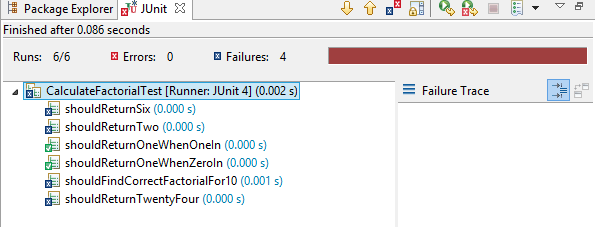
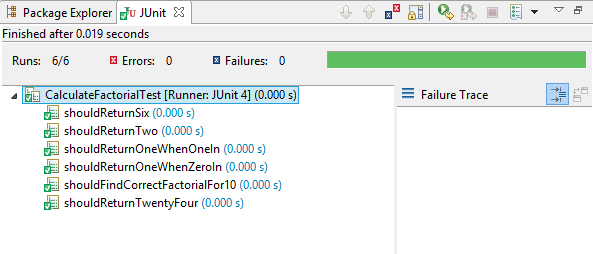